If you have already integrated the SDK and started it, please visit Using the SDK and choose a topic.
Integrating the SDK
Requirements:
- None
Supported Platforms:
- Web (Browser)
Data Privacy:
Integration:
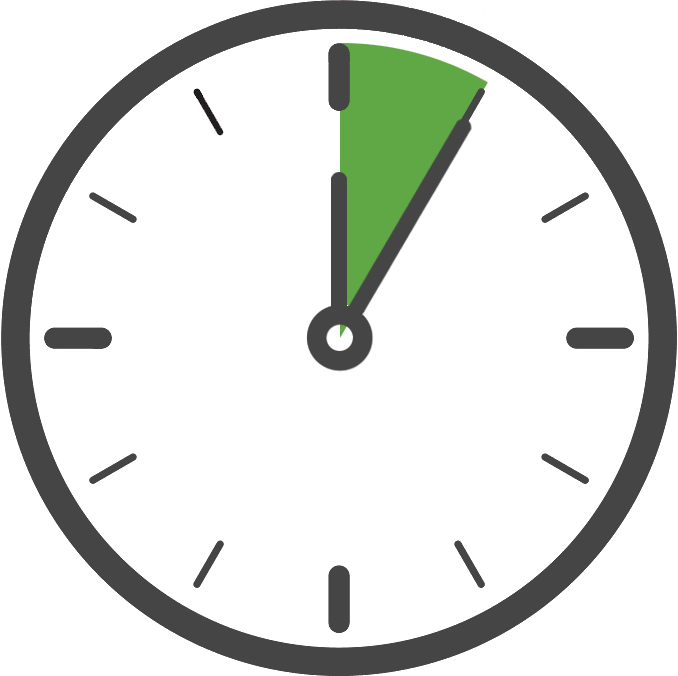
- Copy the Code Snippet
Paste the following code into the head of the site:
function loadScript() {
if (window.kochava) {
console.log("Kochava snippet already included");
return
}
const scriptURL = "https://storage.googleapis.com/kochava-web-assets/kochava.js/v3/kochava.min.js";
const kochavaScript = document.createElement("script");
kochavaScript.type = "text/javascript";
// To disable caching, change this value to true
const disableCaching = false;
kochavaScript.src =
(disableCaching) ? scriptURL + "?c=" + Math.random() : scriptURL;
kochavaScript.async = true;
kochavaScript.onload = () => {
// YOUR CODE HERE
};
document.head.appendChild(kochavaScript);
}
loadScript();
Starting the SDK
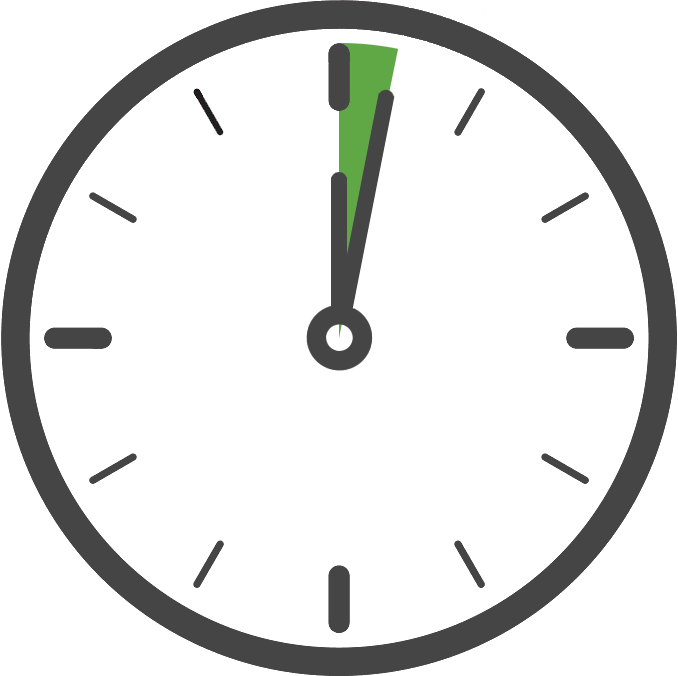
Once you have added the Kochava SDK to your project, the next step is to create and start the SDK class in code. Only your App GUID is required to start the SDK with the default settings, which is the case for typical integrations.
Kochava recommends starting the SDK as soon as the application starts, although this can be done later if needed. Starting the tracker as early as possible will ensure it is started before use, resulting in more accurate data/behavior.
Where your web page starts:
- (Optional) Make any desired pre-start configuration calls (registerIdentityLink, disableAutoPage, useCookies etc).
- Call startWithAppGuid using a valid Kochava App GUID.
Edit the snippet you included earlier to the following:
function loadScript() {
if (window.kochava) {
console.log("Kochava snippet already included");
return
}
const scriptURL = "https://storage.googleapis.com/kochava-web-assets/kochava.js/v3/kochava.min.js";
const kochavaScript = document.createElement("script");
kochavaScript.type = "text/javascript";
// To disable caching, change this value to true
const disableCaching = false;
kochavaScript.src =
(disableCaching) ? scriptURL + "?c=" + Math.random() : scriptURL;
kochavaScript.async = true;
kochavaScript.onload = () => {
// ADD THE START CALL HERE
window.kochava.startWithAppGuid("YOUR_APP_GUID");
};
document.head.appendChild(kochavaScript);
}
loadScript();
Optional Configuration
From here on, the SDK is integrated and ready, the following configuration calls are optional, and are only for special desired SDK behavior. The following code snippets should be placed at in the above snippet, at the comment labeled Optional pre-start calls will go here
.
Call this function with an argument of true to stop the SDK from automatically signaling a page event when the SDK starts.
function loadScript() {
if (window.kochava) {
console.log("Kochava snippet already included");
return
}
const scriptURL = "https://storage.googleapis.com/kochava-web-assets/kochava.js/v3/kochava.min.js";
const kochavaScript = document.createElement("script");
kochavaScript.type = "text/javascript";
// To disable caching, change this value to true
const disableCaching = false;
kochavaScript.src =
(disableCaching) ? scriptURL + "?c=" + Math.random() : scriptURL;
kochavaScript.async = true;
kochavaScript.onload = () => {
// Auto pages will be sent (default)
window.kochava.disableAutoPage(false);
// Auto pages will not be sent
window.kochava.disableAutoPage(true);
window.kochava.startWithAppGuid("YOUR_APP_GUID");
};
document.head.appendChild(kochavaScript);
}
loadScript();
Call this function with an argument of true to drop the Cookie on the website to track a device across sub-domains.
function loadScript() {
if (window.kochava) {
console.log("Kochava snippet already included");
return
}
const scriptURL = "https://storage.googleapis.com/kochava-web-assets/kochava.js/v3/kochava.min.js";
const kochavaScript = document.createElement("script");
kochavaScript.type = "text/javascript";
// To disable caching, change this value to true
const disableCaching = false;
kochavaScript.src =
(disableCaching) ? scriptURL + "?c=" + Math.random() : scriptURL;
kochavaScript.async = true;
kochavaScript.onload = () => {
// Will not use cookies (default)
window.kochava.useCookies(false);
// Will use cookies
window.kochava.useCookies(true);
window.kochava.startWithAppGuid("YOUR_APP_GUID");
};
document.head.appendChild(kochavaScript);
}
loadScript();
If you wish to disable caching of the SDK script, set the disableCaching variable to true.
function loadScript() {
if (window.kochava) {
console.log("Kochava snippet already included");
return
}
const scriptURL = "https://storage.googleapis.com/kochava-web-assets/kochava.js/v3/kochava.min.js";
const kochavaScript = document.createElement("script");
kochavaScript.type = "text/javascript";
// To disable caching, make sure this value is true
const disableCaching = true;
kochavaScript.src =
(disableCaching) ? scriptURL + "?c=" + Math.random() : scriptURL;
kochavaScript.async = true;
kochavaScript.onload = () => {
window.kochava.startWithAppGuid("YOUR_APP_GUID");
};
document.head.appendChild(kochavaScript);
}
loadScript();
Confirm the Integration
After integrating the SDK and adding the code to start the measurement client, launch and run the app for at least 10 seconds or more. During this time the client will start and send an install payload to Kochava. To confirm integration was successful, visit the app’s Install Feed Validation page Apps & Assets > Install Feed Validation. On this page you should see one of two integration messages, indicating integration success or failure.
Integration Successful:
Along with this message you will also see a variety of data points associated with the device used for testing. At this point your integration is successful and you can proceed to the next step(s).
NOTE: It may take a few minutes for the first install to appear within the Install Feed Validation page. If you do not see this message immediately, you may simply need to wait a few minutes and check again.
Integration Not Complete:
If you encounter this message, please review the integration steps, uninstall and reinstall the app, and check again.
Where to Go From Here:
Now that you have completed integration you are ready to utilize the many features offered by the Kochava SDK. Continue on to Using the SDK and choose a topic.