Integrating the SDK
Requirements:
- Xcode 15.2 or higher
Supported Platforms:
- iOS
- macCatalyst
- macOS
- tvOS
- visionOS
- watchOS
Supported Extensions:
- Messages
- Notification Content
- Notification Service
Data Privacy:
Integration:
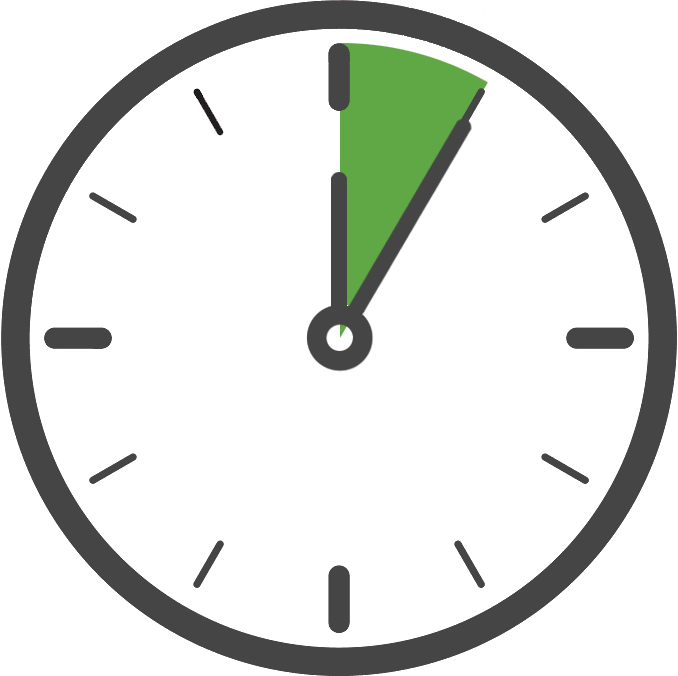
The Kochava SDK is distributed as Swift Packages. A measurement integration includes modules KochavaNetworking and KochavaMeasurement. A tracking integration includes modules KochavaNetworking, KochavaMeasurement, and KochavaTracking.
The tracking module is optional but must be included if you wish to collect the IDFA following ATT authorization. Otherwise, the tracking module can be omitted from your integration and no IDFA collection will take place by the SDK. Previous versions of the SDK automatically included collection of the IDFA, but this functionality is now optional to align with Apple’s privacy manifest requirements.
Module Swift Package Module is Optional
KochavaNetworking
Advanced networking support. A foundational module for the Kochava SDK.https://github.com/Kochava/Apple-SwiftPackage-KochavaNetworking-XCFramework
KochavaMeasurement
Measurement of Attribution and Events.https://github.com/Kochava/Apple-SwiftPackage-KochavaMeasurement-XCFramework
KochavaTracking
Adds tracking features for enhanced advertising measurement. Includes the collection and association of the IDFAhttps://github.com/Kochava/Apple-SwiftPackage-KochavaTracking-XCFramework
✔ How to add a Swift Package:
If this is your first time adding a Swift Package, follow these steps for each of the modules above you wish to add:
- Copy the URL of the swift package to your pasteboard.
- In Xcode 12.2 or higher, go to File > Swift Packages > Add Package Dependency.
- Paste in the URL of the swift package into the prompt.
- Configure the version to the desired major version. Currently you should use
.
At this point the Kochava SDK integration is complete! When viewing code samples throughout our support site, always choose either [Swift] or [Objective-C] code samples.
NOTE: It is important to sometimes perform a clean build when building for a new version of our SDK (Command – Shift – K). Apple’s package management system does not always automatically recognize that the packages have been updated
SDK NOTE: With the release of version 8.0.0 of the Kochava Apple SDK, we discontinued cocoapods distribution in favor of Swift Packages. Please contact support@kochava.com if you have any questions or concerns. Module Cocoapod Module is Optional
KochavaTracker
Attribution, event tracking, and analytics.Apple-Cocoapod-KochavaTracker
KochavaAdNetwork (v5)
Support for SKAdNetwork. This module is required for integrations which intend to leverage Apple’s SKAdNetwork support.
This optional module was moved into module KochavaTracker in v6 and does not need to be added separately in newer versions.Apple-Cocoapod-KochavaAdNetwork
✔ How to add a Cocoapod:
If this is your first time adding a Cocoapod, follow these steps for each of the modules above you wish to add:
- Open your Podfile in an editor. If you do not have a Podfile yet, see Using Cocoapods.
- Add the module, and configure the version to the desired major version. Currently you should use 7.
Example:
target 'MyApp' do pod 'Apple-Cocoapod-KochavaTracker', '~> 7.0' end
Sample Code:
XCFramework(s), Swift —
import KochavaTracker
XCFramework(s), Objective-C —
@import KochavaTracker;
At this point the v5 SDK integration is complete! When viewing code samples throughout our support site, always choose either Swift or Objective-C code samples.
SDK NOTE: With the release of version 8.0.0 of the Kochava Apple SDK, we discontinued all xcframework standalone repositories in favor of Swift Packages. The Swift Package repositories already contain these same xcframeworks. Please contact support@kochava.com if you have any questions or concerns. Module XCFramework Module is Optional
KochavaCore
Core SDK functionality.KochavaTracker
Attribution, event tracking, and analytics.KochavaAdNetwork (v5)
Support for SKAdNetwork. This module is required for integrations which intend to leverage Apple’s SKAdNetwork support.
This optional module was moved into module KochavaTracker in v6 and does not need to be added separately in newer versions.✔ How to add an XCFramework:
Follow these steps for each of the modules above you wish to add:
- Download the XCFramework from the link above and add it to your project.
- Download the release archive containing the XCFramework.
- Open the archive and copy the XCFramework into your Xcode project folder using Finder. You will typically want to add this file to the Frameworks folder of your project.
- Right click on your project in Xcode, select Add Files to (project name), and add the included file to your project.
- If you are working with multiple targets, you will want to ensure that you have included the XCFramework in each of the desired targets by selecting the file and reviewing its target membership in the inspector, which appears on the right side in Xcode.
- Ensure that the XCFramework is included in Frameworks, Libraries, and Embedded Content in your project target (located under the General tab). It will normally have been added at the moment you added the file to your target.
- Import the XCFramework.
XCFramework Addition Details:
Expand for Details
Sample Code:
XCFramework(s), Swift —
import KochavaTracker
XCFramework(s), Objective-C —
@import KochavaTracker;
Starting the Measurement Client
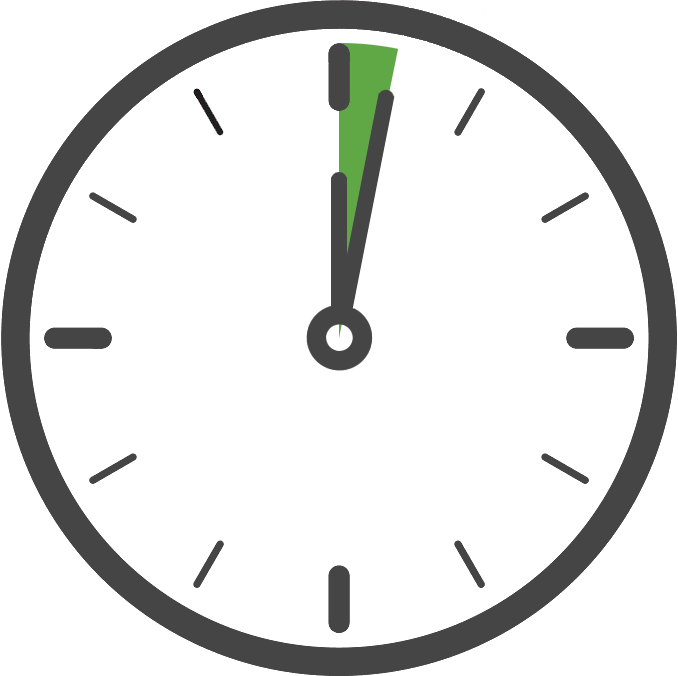
Once you have added the Kochava SDK to your project, the next step is to configure and start the measurement client. Only your App GUID is required to start the client with the default settings, which is the case for typical integrations.
We recommend starting the client in the method within your AppDelegate that is called when your application did finish launching, although it can be done elsewhere if needed. Starting the client as early as possible will provide more accurate session reporting and help to ensure the client is actively processing tasks when subsequent requests are made. The call to start the measurement client should be made one time when your application launches.
Start the Shared Instance:
import KochavaNetworking import KochavaMeasurement import KochavaTracking // optional func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { Log.shared.level = .info let measurement = Measurement.shared measurement.start(appGUIDString: "_YOUR_APP_GUID_") return true }
@import KochavaNetworking; @import KochavaMeasurement; @import KochavaTracking; // optional - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { KVALog.shared.level = KVALog_Level.info; KVAMeasurement *measurement = KVAMeasurement.shared; [measurement startWithAppGUIDString:@"_YOUR_APP_GUID_"]; return YES; }
import KochavaTracker func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { KVATracker.shared.start(withAppGUIDString: "_YOUR_APP_GUID_") return true }
Confirm the Integration
After integrating the SDK and adding the code to start the measurement client, launch and run the app for at least 10 seconds or more. During this time the client will start and send an install payload to Kochava. To confirm integration was successful, visit the app’s Install Feed Validation page Apps & Assets > Install Feed Validation. On this page you should see one of two integration messages, indicating integration success or failure.
Integration Successful:
Along with this message you will also see a variety of data points associated with the device used for testing. At this point your integration is successful and you can proceed to the next step(s).
NOTE: It may take a few minutes for the first install to appear within the Install Feed Validation page. If you do not see this message immediately, you may simply need to wait a few minutes and check again.
Integration Not Complete:
If you encounter this message, please review the integration steps, uninstall and reinstall the app, and check again.
Where to Go From Here:
Now that you have completed integration you are ready to utilize the many features offered by the Kochava SDK. Continue on to Using the SDK and choose a topic.